Build, Deploy, Utilize and Run
In this section you will learn the paradigms to use Drools library as part of KIE (Knowledge Is Everything) for Building, Deploying and Running your Drools-based solutions.
Introduction
Drools since version 6.0
introduced a new configuration and convention approach to building KIE bases, instead of using the programmatic builder approach of the 5.x
series.
Building Drools-based KIE project make use of Maven, and aligns with Maven practices. A KIE project or module is simply a Maven Java project or module; with an additional metadata file META-INF/kmodule.xml. The kmodule.xml file is the descriptor that selects resources to KIE bases and configures those KIE bases and sessions.
While standard Maven can build and package KIE resources, it will not provide validation at build time.
There is a Maven plugin (kie-maven-plugin
) which is recommended to use to get build time validation.
The plugin also generates many classes, making the runtime loading faster too.
The example project layout and Maven POM descriptor is illustrated in the screenshot
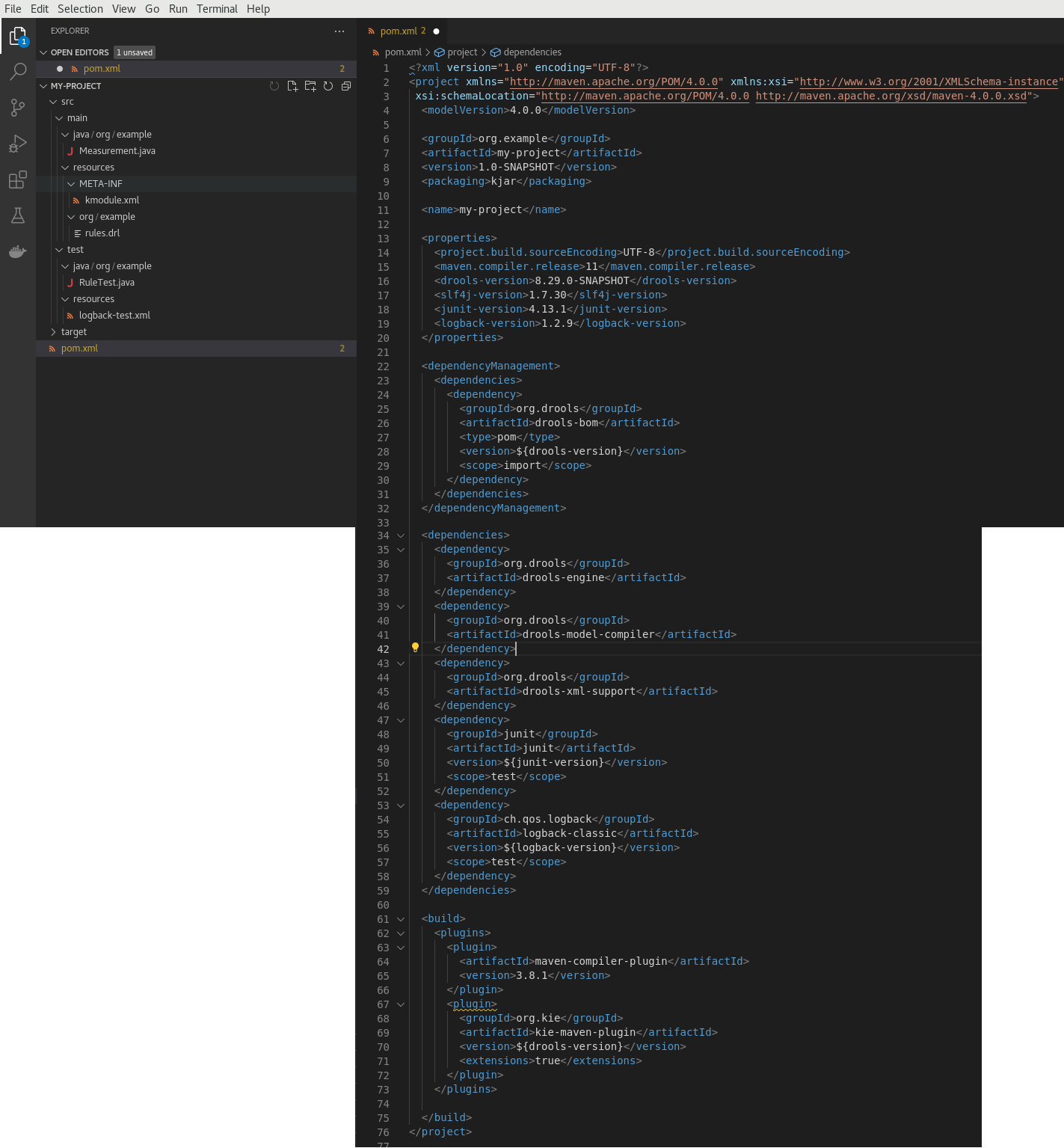
KIE uses defaults to minimise the amount of configuration. With an empty kmodule.xml being the simplest configuration. There must always be a kmodule.xml file, even if empty, as it’s used for discovery of the JAR and its contents.
Maven can either 'mvn install' to deploy a KieModule to the local machine, where all other applications on the local machine use it. Or it can 'mvn deploy' to push the KieModule to a remote Maven repository. Building the Application will pull in the KieModule and populate the local Maven repository in the process.
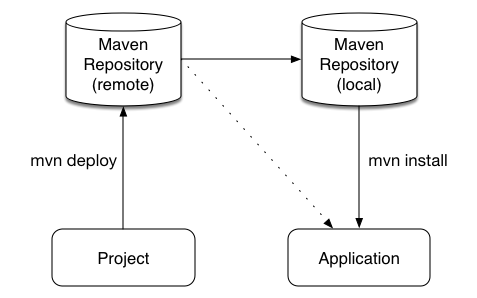
JARs can be deployed in one of two ways. Either added to the classpath, like any other JAR in a Maven dependency listing, or they can be dynamically loaded at runtime. KIE will scan the classpath to find all the JARs with a kmodule.xml in it. Each found JAR is represented by the KieModule interface. The terms classpath KieModule and dynamic KieModule are used to refer to the two loading approaches. While dynamic modules support side by side versioning, classpath modules do not. Further once a module is on the classpath, no other version may be loaded dynamically.
Detailed references for the API are included in the next sections, the impatient can jump straight to the examples section, which is fairly self-explanatory on the different use cases.
Building
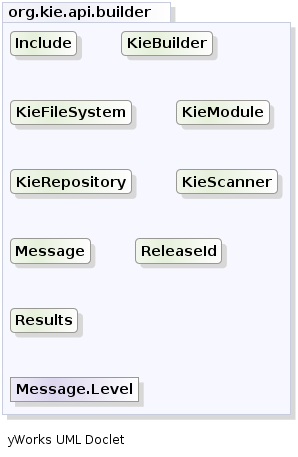
Creating and building a Kie Project
A Kie Project has the structure of a normal Maven project with the only peculiarity of including a kmodule.xml file defining in a declaratively way the KieBase
s and KieSession
s that can be created from it.
This file has to be placed in the resources/META-INF folder of the Maven project while all the other Kie artifacts, such as DRL or Excel files, must be stored in the resources folder or in any other subfolder under it.
Since meaningful defaults have been provided for all configuration aspects, the simplest kmodule.xml file can contain just an empty kmodule tag like the following:
<?xml version="1.0" encoding="UTF-8"?>
<kmodule xmlns="http://www.drools.org/xsd/kmodule"/>
In this way the kmodule will contain one single default KieBase
.
All Kie assets stored under the resources folder, or any of its subfolders, will be compiled and added to it.
To trigger the building of these artifacts it is enough to create a KieContainer
for them.
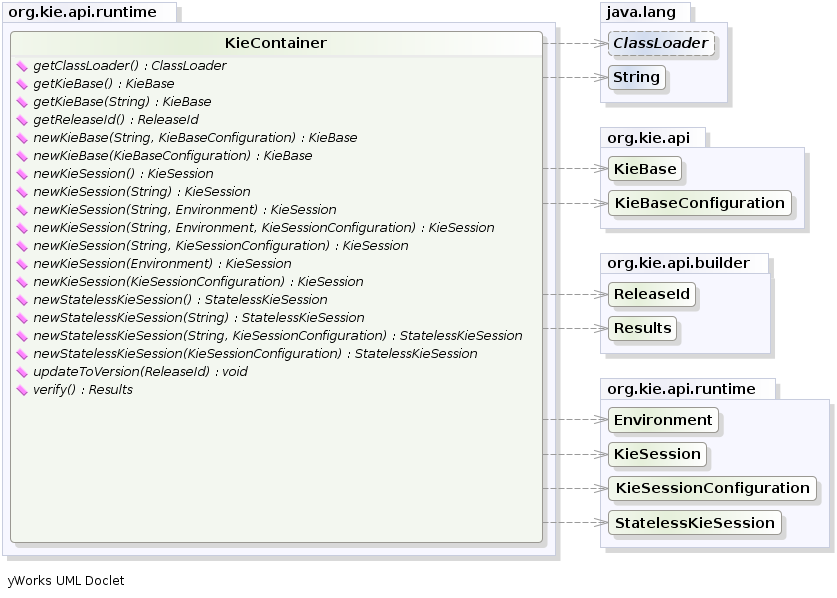
For this simple case it is enough to create a KieContainer
that reads the files to be built from the classpath:
KieServices kieServices = KieServices.Factory.get();
KieContainer kContainer = kieServices.getKieClasspathContainer();
KieServices
is the interface from where it possible to access all the Kie building and runtime facilities:
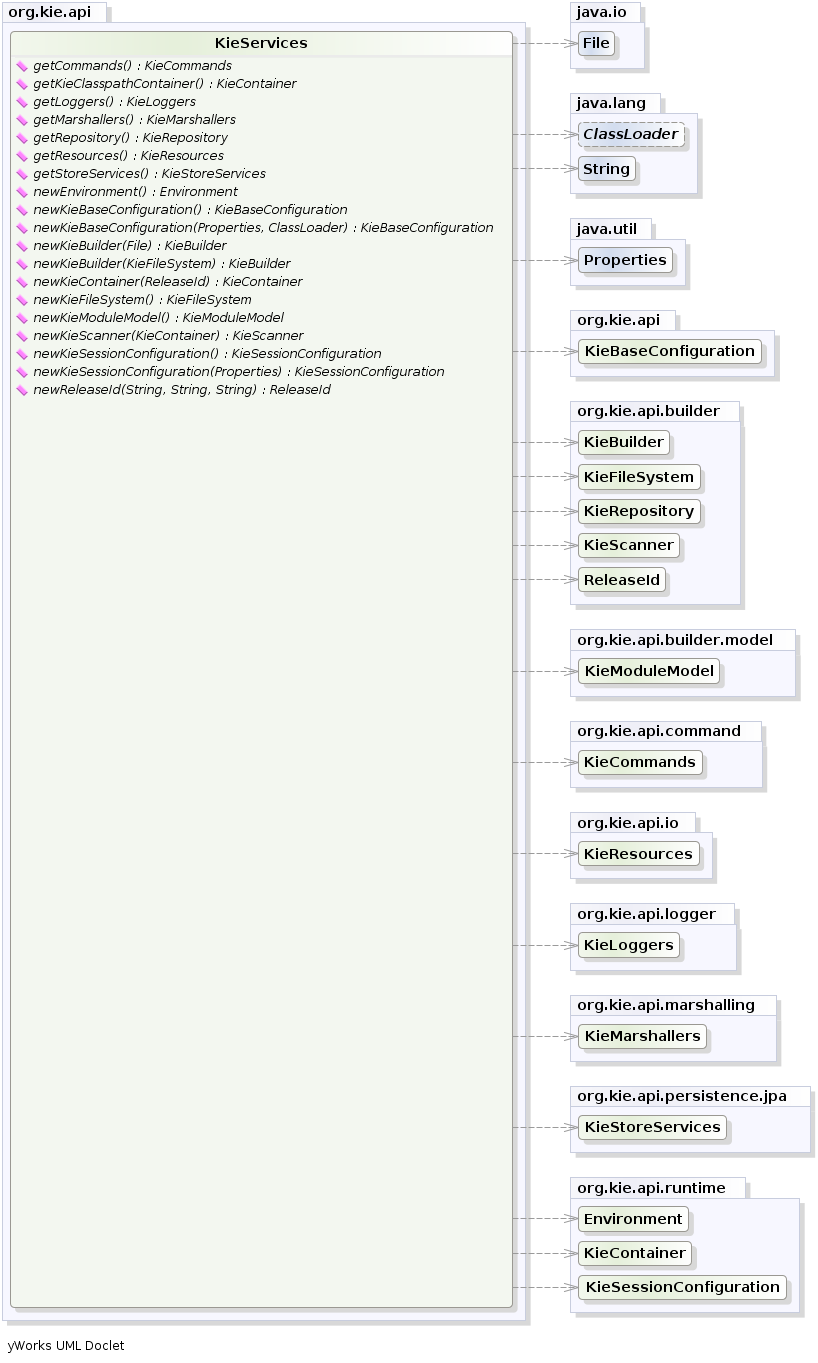
In this way all the Java sources and the Kie resources are compiled and deployed into the KieContainer which makes its contents available for use at runtime.
The kmodule.xml file
As explained in the former section, the kmodule.xml file is the place where it is possible to declaratively configure the KieBase
(s) and KieSession
(s) that can be created from a KIE project.
In particular a KieBase
is a repository of all the application’s knowledge definitions.
It will contain rules, processes, functions, and type models.
The KieBase
itself does not contain data; instead, sessions are created from the KieBase
into which data can be inserted and from which process instances may be started.
Creating the KieBase
can be heavy, whereas session creation is very light, so it is recommended that KieBase
be cached where possible to allow for repeated session creation.
However end-users usually shouldn’t worry about it, because this caching mechanism is already automatically provided by the KieContainer
.
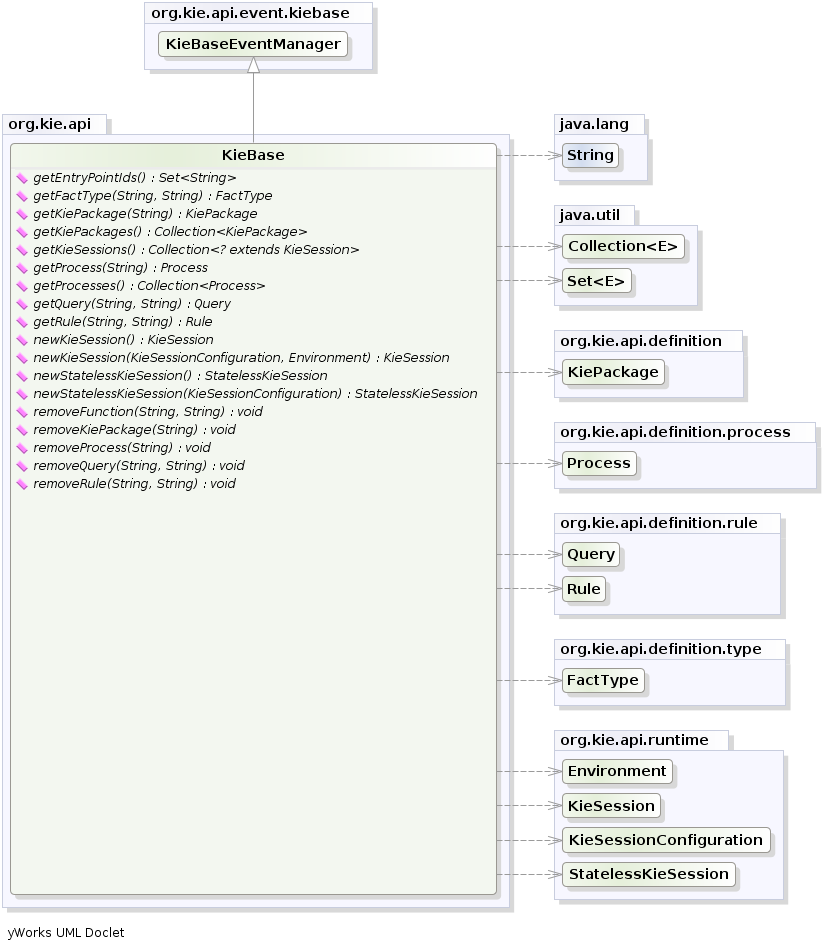
Conversely the KieSession
stores and executes on the runtime data.
It is created from the KieBase
or more easily can be created directly from the KieContainer
if it has been defined in the kmodule.xml file
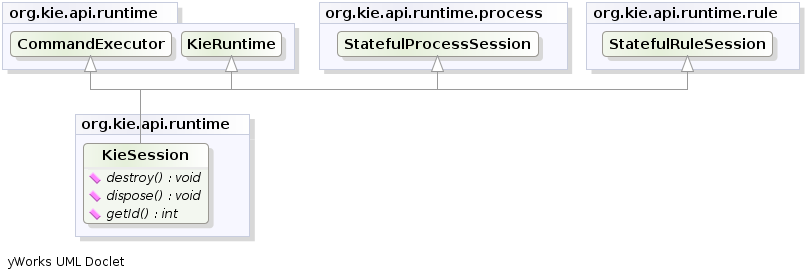
The kmodule.xml allows to define and configure one or more KieBase
s and for each KieBase
all the different KieSession
s that can be created from it, as showed by the follwing example:
<kmodule xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://www.drools.org/xsd/kmodule">
<configuration>
<property key="drools.evaluator.supersetOf" value="org.mycompany.SupersetOfEvaluatorDefinition"/>
</configuration>
<kbase name="KBase1" default="true" eventProcessingMode="cloud" equalsBehavior="equality" declarativeAgenda="enabled" packages="org.domain.pkg1">
<ksession name="KSession2_1" type="stateful" default="true"/>
<ksession name="KSession2_2" type="stateless" default="false" beliefSystem="jtms"/>
</kbase>
<kbase name="KBase2" default="false" eventProcessingMode="stream" equalsBehavior="equality" declarativeAgenda="enabled" packages="org.domain.pkg2, org.domain.pkg3" includes="KBase1">
<ksession name="KSession3_1" type="stateful" default="false" clockType="realtime">
<fileLogger file="drools.log" threaded="true" interval="10"/>
<workItemHandlers>
<workItemHandler name="name" type="org.domain.WorkItemHandler"/>
</workItemHandlers>
<calendars>
<calendar name="monday" type="org.domain.Monday"/>
</calendars>
<listeners>
<ruleRuntimeEventListener type="org.domain.RuleRuntimeListener"/>
<agendaEventListener type="org.domain.FirstAgendaListener"/>
<agendaEventListener type="org.domain.SecondAgendaListener"/>
<processEventListener type="org.domain.ProcessListener"/>
</listeners>
</ksession>
</kbase>
</kmodule>
Here the
tag contains a list of key-value pairs that are the optional properties used to configure the KieBase
s building process.
For instance this sample kmodule.xml file defines an additional custom operator named supersetOf
and implemented by the org.mycompany.SupersetOfEvaluatorDefinition
class.
After this 2 KieBase
s have been defined and it is possible to instance 2 different types of KieSession
s from the first one, while only one from the second.
A list of the attributes that can be defined on the kbase tag, together with their meaning and default values follows:
Attribute name | Default value | Admitted values | Meaning |
---|---|---|---|
name |
none |
any |
The name with which retrieve this KieBase from the KieContainer. This is the only mandatory attribute. |
includes |
none |
any comma separated list |
A comma separated list of other KieBases contained in this kmodule. The artifacts of all these KieBases will be also included in this one. |
packages |
all |
any comma separated list |
By default all the Drools artifacts under the resources folder, at any level, are included into the KieBase. This attribute allows to limit the artifacts that will be compiled in this KieBase to only the ones belonging to the list of packages. |
default |
false |
true, false |
Defines if this KieBase is the default one for this module, so it can be created from the KieContainer without passing any name to it. There can be at most one default KieBase in each module. |
equalsBehavior |
identity |
identity, equality |
Defines the behavior of Drools when a new fact is inserted into the Working Memory. With identity it always create a new FactHandle unless the same object isn’t already present in the Working Memory, while with equality only if the newly inserted object is not equal (according to its equal method) to an already existing fact. |
eventProcessingMode |
cloud |
cloud, stream |
When compiled in cloud mode the KieBase treats events as normal facts, while in stream mode allow temporal reasoning on them. |
declarativeAgenda |
disabled |
disabled, enabled |
Defines if the Declarative Agenda is enabled or not. |
Similarly all attributes of the ksession tag (except of course the name) have meaningful default. They are listed and described in the following table:
Attribute name | Default value | Admitted values | Meaning |
---|---|---|---|
name |
none |
any |
Unique name of this KieSession. Used to fetch the KieSession from the KieContainer. This is the only mandatory attribute. |
type |
stateful |
stateful, stateless |
A stateful session allows to iteratively work with the Working Memory, while a stateless one is a one-off execution of a Working Memory with a provided data set. |
default |
false |
true, false |
Defines if this KieSession is the default one for this module, so it can be created from the KieContainer without passing any name to it. In each module there can be at most one default KieSession for each type. |
clockType |
realtime |
realtime, pseudo |
Defines if events timestamps are determined by the system clock or by a pseudo clock controlled by the application. This clock is especially useful for unit testing temporal rules. |
beliefSystem |
simple |
simple, jtms, defeasible |
Defines the type of belief system used by the KieSession. |
As outlined in the former kmodule.xml sample, it is also possible to declaratively create on each KieSession
a file (or a console) logger, one or more WorkItemHandler
s and Calendar
s plus some listeners that can be of 3 different types: ruleRuntimeEventListener, agendaEventListener and processEventListener
Having defined a kmodule.xml like the one in the former sample, it is now possible to simply retrieve the KieBases and KieSessions from the KieContainer using their names.
KieServices kieServices = KieServices.Factory.get();
KieContainer kContainer = kieServices.getKieClasspathContainer();
KieBase kBase1 = kContainer.getKieBase("KBase1");
KieSession kieSession1 = kContainer.newKieSession("KSession2_1");
StatelessKieSession kieSession2 = kContainer.newStatelessKieSession("KSession2_2");
It has to be noted that since KSession2_1 and KSession2_2 are of 2 different types (the first is stateful, while the second is stateless) it is necessary to invoke 2 different methods on the KieContainer
according to their declared type.
If the type of the KieSession
requested to the KieContainer
doesn’t correspond with the one declared in the kmodule.xml file the KieContainer
will throw a RuntimeException
.
Also since a KieBase
and a KieSession
have been flagged as default is it possible to get them from the KieContainer
without passing any name.
KieContainer kContainer = ...
KieBase kBase1 = kContainer.getKieBase(); // returns KBase1
KieSession kieSession1 = kContainer.newKieSession(); // returns KSession2_1
Since a Kie project is also a Maven project the groupId, artifactId and version declared in the pom.xml file are used to generate a ReleaseId
that uniquely identifies this project inside your application.
This allows creation of a new KieContainer from the project by simply passing its ReleaseId
to the KieServices
.
KieServices kieServices = KieServices.Factory.get();
ReleaseId releaseId = kieServices.newReleaseId( "org.acme", "myartifact", "1.0" );
KieContainer kieContainer = kieServices.newKieContainer( releaseId );
KieBase and KiePackage don’t support serialization since Drools 6. You need to build KieBase through KieContainer. On the other hand, KieSession can be marshalled/unmarshalled by KieMashaller. See Marshalling. |
Building with Maven
The KIE plugin for Maven ensures that artifact resources are validated and pre-compiled, it is recommended that this is used at all times.
To use the plugin simply add it to the build section of the Maven pom.xml and activate it by using packaging kjar
.
<packaging>kjar</packaging>
...
<build>
<plugins>
<plugin>
<groupId>org.kie</groupId>
<artifactId>kie-maven-plugin</artifactId>
<version>${version.org.drools}</version>
<extensions>true</extensions>
</plugin>
</plugins>
</build>
The plugin comes with support for all the Drools/jBPM knowledge resources.
However, in case you are using specific KIE annotations in your Java classes, like for example @kie.api.Position
, you will need to add compile time dependency on kie-api
into your project.
We recommend to use the provided scope for all the additional KIE dependencies.
That way the kjar stays as lightweight as possible, and not dependant on any particular KIE version.
Building a KIE module without the Maven plugin will copy all the resources, as is, into the resulting JAR. When that JAR is loaded by the runtime, it will attempt to build all the resources then. If there are compilation issues it will return a null KieContainer. It also pushes the compilation overhead to the runtime. In general this is not recommended, and the Maven plugin should always be used.
Engine dependency
Drools has 3 types of engine dependency that aggregate a required collection of dependencies.
drools-ruleunits-engine
is the standard engine for rule units that enables an executable model.
drools-engine
is the standard engine for traditional DRL syntax that enables an executable model.
drools-engine-classic
is the old engine that utilizes an MVEL interpreter. The drools-engine-classic
and drools-mvel
are now deprecated, so use drools-ruleunits-engine
or drools-engine
instead.
<dependency>
<groupId>org.drools</groupId>
<artifactId>drools-engine</artifactId>
</dependency>
For example:
|
Defining a KieModule programmatically
It is also possible to define the KieBase
s and KieSession
s belonging to a KieModule programmatically instead of the declarative definition in the kmodule.xml file.
The same programmatic API also allows in explicitly adding the file containing the Kie artifacts instead of automatically read them from the resources folder of your project.
To do that it is necessary to create a KieFileSystem
, a sort of virtual file system, and add all the resources contained in your project to it.
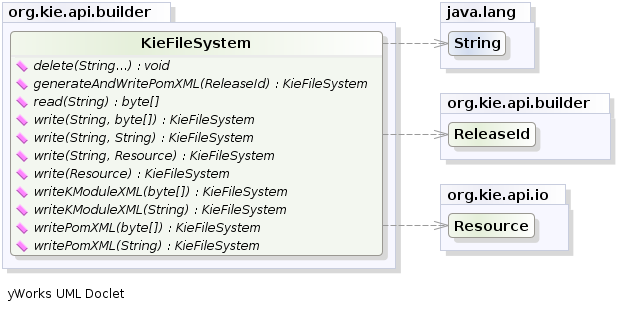
Like all other Kie core components you can obtain an instance of the KieFileSystem
from the KieServices
.
The kmodule.xml configuration file must be added to the filesystem.
This is a mandatory step.
Kie also provides a convenient fluent API, implemented by the KieModuleModel
, to programmatically create this file.
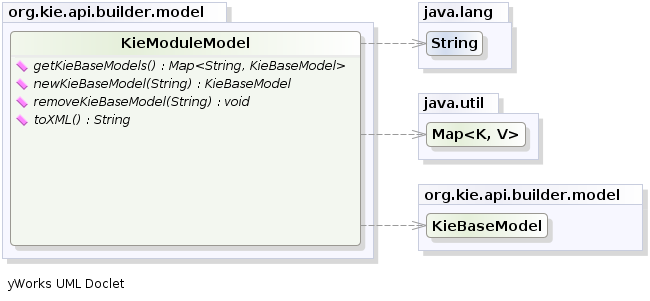
To do this in practice it is necessary to create a KieModuleModel
from the KieServices
, configure it with the desired KieBase
s and KieSession
s, convert it in XML and add the XML to the KieFileSystem
.
This process is shown by the following example:
KieServices kieServices = KieServices.Factory.get();
KieModuleModel kieModuleModel = kieServices.newKieModuleModel();
KieBaseModel kieBaseModel1 = kieModuleModel.newKieBaseModel( "KBase1 ")
.setDefault( true )
.setEqualsBehavior( EqualityBehaviorOption.EQUALITY )
.setEventProcessingMode( EventProcessingOption.STREAM );
KieSessionModel ksessionModel1 = kieBaseModel1.newKieSessionModel( "KSession1" )
.setDefault( true )
.setType( KieSessionModel.KieSessionType.STATEFUL )
.setClockType( ClockTypeOption.get("realtime") );
KieFileSystem kfs = kieServices.newKieFileSystem();
kfs.writeKModuleXML(kieModuleModel.toXML());
At this point it is also necessary to add to the KieFileSystem
, through its fluent API, all others Kie artifacts composing your project.
These artifacts have to be added in the same position of a corresponding usual Maven project.
KieFileSystem kfs = ...
kfs.write( "src/main/resources/KBase1/ruleSet1.drl", stringContainingAValidDRL )
.write( "src/main/resources/dtable.xls",
kieServices.getResources().newInputStreamResource( dtableFileStream ) );
This example shows that it is possible to add the Kie artifacts both as plain Strings and as Resource
s.
In the latter case the Resource
s can be created by the KieResources
factory, also provided by the KieServices
.
The KieResources
provides many convenient factory methods to convert an InputStream
, a File
, or a String
representing a path of your file system to a Resource
that can be managed by the KieFileSystem
.
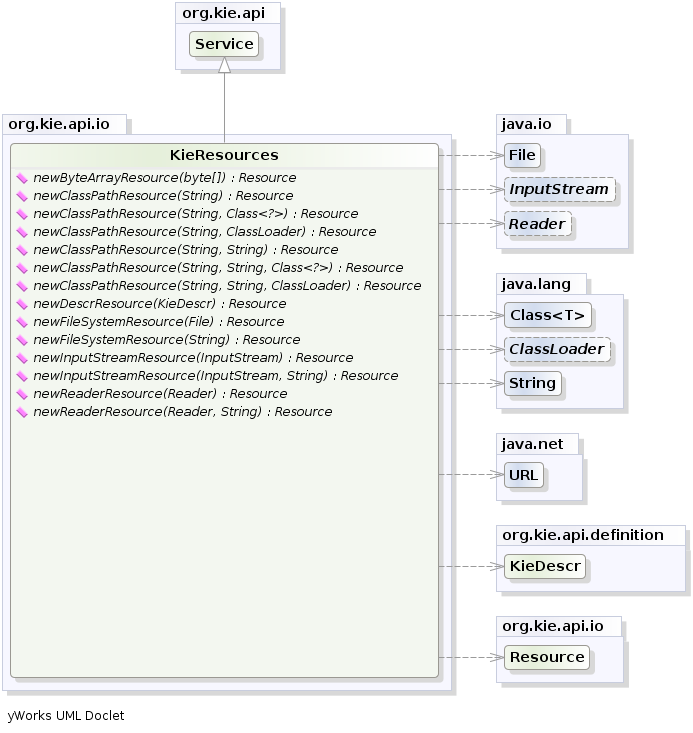
URLResource is no longer available with Drools 8 series, to ensure a more reproducible knowledge base build (avoiding remote URLs) and for better security. If you used URLResource in the past, you can manage the local-fetch of the remote resource externally to your Drools application, so to limit Drools build concerns to local resources containing knowledge assets.
|
Normally the type of a Resource
can be inferred from the extension of the name used to add it to the KieFileSystem
.
However it also possible to not follow the Kie conventions about file extensions and explicitly assign a specific ResourceType
to a Resource
as shown below:
KieFileSystem kfs = ...
kfs.write( "src/main/resources/myDrl.txt",
kieServices.getResources().newInputStreamResource( drlStream )
.setResourceType(ResourceType.DRL) );
Add all the resources to the KieFileSystem
and build it by passing the KieFileSystem
to a KieBuilder
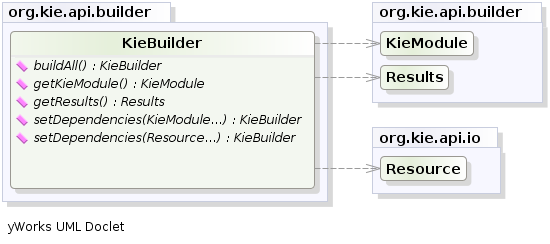
When the contents of a KieFileSystem
are successfully built, the resulting KieModule
is automatically added to the KieRepository
.
The KieRepository
is a singleton acting as a repository for all the available KieModule
s.
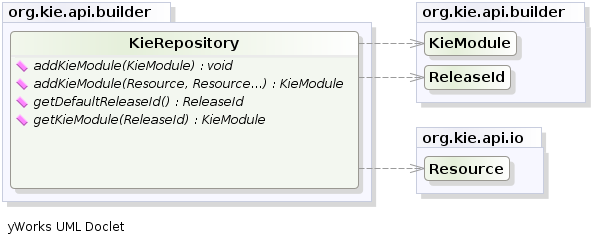
After this it is possible to create through the KieServices
a new KieContainer
for that KieModule
using its ReleaseId
.
However, since in this case the KieFileSystem
doesn’t contain any pom.xml file (it is possible to add one using the KieFileSystem.writePomXML
method), Kie cannot determine the ReleaseId
of the KieModule
and assign to it a default one.
This default ReleaseId
can be obtained from the KieRepository
and used to identify the KieModule
inside the KieRepository
itself.
The following example shows this whole process.
KieServices kieServices = KieServices.Factory.get();
KieFileSystem kfs = ...
kieServices.newKieBuilder( kfs ).buildAll();
KieContainer kieContainer = kieServices.newKieContainer(kieServices.getRepository().getDefaultReleaseId());
At this point it is possible to get KieBase
s and create new KieSession
s from this KieContainer
exactly in the same way as in the case of a KieContainer
created directly from the classpath.
It is a best practice to check the compilation results.
The KieBuilder
reports compilation results of 3 different severities: ERROR, WARNING and INFO.
An ERROR indicates that the compilation of the project failed and in the case no KieModule
is produced and nothing is added to the KieRepository
.
WARNING and INFO results can be ignored, but are available for inspection.
KieBuilder kieBuilder = kieServices.newKieBuilder( kfs ).buildAll();
assertEquals( 0, kieBuilder.getResults().getMessages( Message.Level.ERROR ).size() );
Changing the Default Build Result Severity
In some cases, it is possible to change the default severity of a type of build result. For instance, when a new rule with the same name of an existing rule is added to a package, the default behavior is to replace the old rule by the new rule and report it as an INFO. This is probably ideal for most use cases, but in some deployments the user might want to prevent the rule update and report it as an error.
Changing the default severity for a result type, configured like any other option in Drools, can be done by API calls, system properties or configuration files. As of this version, Drools supports configurable result severity for rule updates and function updates. To configure it using system properties or configuration files, the user has to use the following properties:
// sets the severity of rule updates
drools.kbuilder.severity.duplicateRule = <INFO|WARNING|ERROR>
// sets the severity of function updates
drools.kbuilder.severity.duplicateFunction = <INFO|WARNING|ERROR>
Deploying
KieBase
The KieBase
is a repository of all the application’s knowledge definitions.
It will contain rules, processes, functions, and type models.
The KieBase
itself does not contain data; instead, sessions are created from the KieBase
into which data can be inserted and from which process instances may be started.
The KieBase
can be obtained from the KieContainer
containing the KieModule
where the KieBase
has been defined.
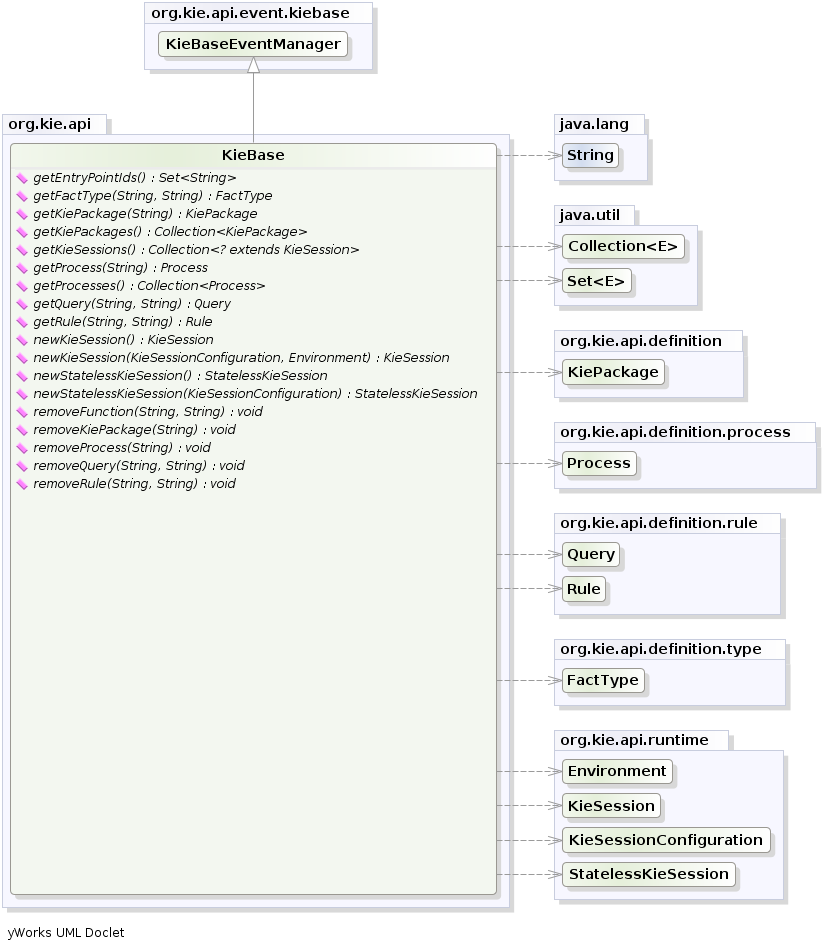
When the KieBase
needs to resolve types that are not in the default class loader, it will be necessary to create a KieBaseConfiguration
with an additional class loader and pass it to KieContainer
when creating a new KieBase
from it.
KieServices kieServices = KieServices.Factory.get();
KieBaseConfiguration kbaseConf = kieServices.newKieBaseConfiguration( null, MyType.class.getClassLoader() );
KieBase kbase = kieContainer.newKieBase( kbaseConf );
KieSessions and KieBase Modifications
KieSessions will be discussed in more detail in section "Running". The KieBase
creates and returns KieSession
objects, and it may optionally keep references to those.
When KieBase
modifications occur those modifications are applied against the data in the sessions.
This reference is a weak reference and it is also optional, which is controlled by a boolean flag.
KieScanner
The KieScanner
allows continuous monitoring of your Maven repository to check whether a new release of a Kie project has been installed.
A new release is deployed in the KieContainer
wrapping that project.
The use of the KieScanner
requires kie-ci.jar to be on the classpath.
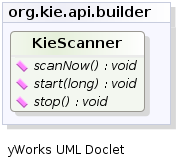
A KieScanner
can be registered on a KieContainer
as in the following example.
KieServices kieServices = KieServices.Factory.get();
ReleaseId releaseId = kieServices.newReleaseId( "org.acme", "myartifact", "1.0-SNAPSHOT" );
KieContainer kContainer = kieServices.newKieContainer( releaseId );
KieScanner kScanner = kieServices.newKieScanner( kContainer );
// Start the KieScanner polling the Maven repository every 10 seconds
kScanner.start( 10000L );
In this example the KieScanner
is configured to run with a fixed time interval, but it is also possible to run it on demand by invoking the scanNow()
method on it.
If the KieScanner
finds, in the Maven repository, an updated version of the Kie project used by that KieContainer
it automatically downloads the new version and triggers an incremental build of the new project.
At this point, existing KieBase
s and KieSession
s under the control of KieContainer
will get automatically upgraded with it - specifically, those KieBase
s obtained with getKieBase()
along with their related KieSession
s, and any KieSession
obtained directly with KieContainer.newKieSession()
thus referencing the default KieBase
.
Additionally, from this moment on, all the new KieBase
s and KieSession
s created from that KieContainer
will use the new project version.
Please notice however any existing KieBase
which was obtained via newKieBase()
before the KieScanner upgrade, and any of its related KieSession
s, will not get automatically upgraded; this is because KieBase
s obtained via newKieBase()
are not under the direct control of the KieContainer
.
The KieScanner
will only pickup changes to deployed jars if it is using a SNAPSHOT, version range, the LATEST, or the RELEASE setting.
Fixed versions will not automatically update at runtime.
In case you don’t want to install a maven repository, it is also possible to have a KieScanner
that works
by simply fetching update from a folder of a plain file system. You can create such a KieScanner
as simply as
KieServices kieServices = KieServices.Factory.get();
KieScanner kScanner = kieServices.newKieScanner( kContainer, "/myrepo/kjars" );
where "/myrepo/kjars" will be the folder where the KieScanner
will look for kjar updates. The jar files placed in this folder
have to follow the maven convention and then have to be a name in the form {artifactId}-{versionId}.jar
.
Maven Versions and Dependencies
Maven supports a number of mechanisms to manage versioning and dependencies within applications. Modules can be published with specific version numbers, or they can use the SNAPSHOT suffix. Dependencies can specify version ranges to consume, or take advantage of SNAPSHOT mechanism.
StackOverflow provides a very good description for this, which is reproduced below.
Since Maven 3.x metaversions RELEASE and LATEST are no longer supported for the sake of reproducible builds. |
See the POM Syntax section of the Maven book for more details.
Here’s an example illustrating the various options. In the Maven repository, com.foo:my-foo has the following metadata:
<metadata>
<groupId>com.foo</groupId>
<artifactId>my-foo</artifactId>
<version>2.0.0</version>
<versioning>
<release>1.1.1</release>
<versions>
<version>1.0</version>
<version>1.0.1</version>
<version>1.1</version>
<version>1.1.1</version>
<version>2.0.0</version>
</versions>
<lastUpdated>20090722140000</lastUpdated>
</versioning>
</metadata>
If a dependency on that artifact is required, you have the following options (other version ranges can be specified of course, just showing the relevant ones here): Declare an exact version (will always resolve to 1.0.1):
<version>[1.0.1]</version>
Declare an explicit version (will always resolve to 1.0.1 unless a collision occurs, when Maven will select a matching version):
<version>1.0.1</version>
Declare a version range for all 1.x (will currently resolve to 1.1.1):
<version>[1.0.0,2.0.0)</version>
Declare an open-ended version range (will resolve to 2.0.0):
<version>[1.0.0,)</version>
Declare the version as LATEST (will resolve to 2.0.0):
<version>LATEST</version>
Declare the version as RELEASE (will resolve to 1.1.1):
<version>RELEASE</version>
Note that by default your own deployments will update the "latest" entry in the Maven metadata, but to update the "release" entry, you need to activate the "release-profile" from the Maven super POM. You can do this with either "-Prelease-profile" or "-DperformRelease=true"
Settings.xml and Remote Repository Setup
The maven settings.xml is used to configure Maven execution. Detailed instructions can be found at the Maven website:
The settings.xml file can be located in 3 locations, the actual settings used is a merge of those 3 locations.
-
The Maven install:
$M2_HOME/conf/settings.xml
-
A user’s install:
${user.home}/.m2/settings.xml
-
Folder location specified by the system property
kie.maven.settings.custom
The settings.xml is used to specify the location of remote repositories. It is important that you activate the profile that specifies the remote repository, typically this can be done using "activeByDefault":
<profiles>
<profile>
<id>profile-1</id>
<internalMatch>
<activeByDefault>true</activeByDefault>
</internalMatch>
...
</profile>
</profiles>
Maven provides detailed documentation on using multiple remote repositories:
Running
KieBase
The KieBase
is a repository of all the application’s knowledge definitions.
It will contain rules, processes, functions, and type models.
The KieBase
itself does not contain data; instead, sessions are created from the KieBase
into which data can be inserted and from which process instances may be started.
The KieBase
can be obtained from the KieContainer
containing the KieModule
where the KieBase
has been defined.
KieBase kBase = kContainer.getKieBase();
KieSession
The KieSession
stores and executes on the runtime data.
It is created from the KieBase
.
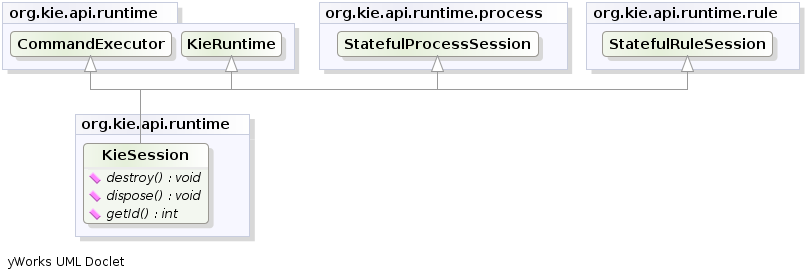
KieSession ksession = kbase.newKieSession();
KieRuntime
KieRuntime
The KieRuntime
provides methods that are applicable to both rules and processes, such as setting globals and registering channels.
("Exit point" is an obsolete synonym for "channel".)
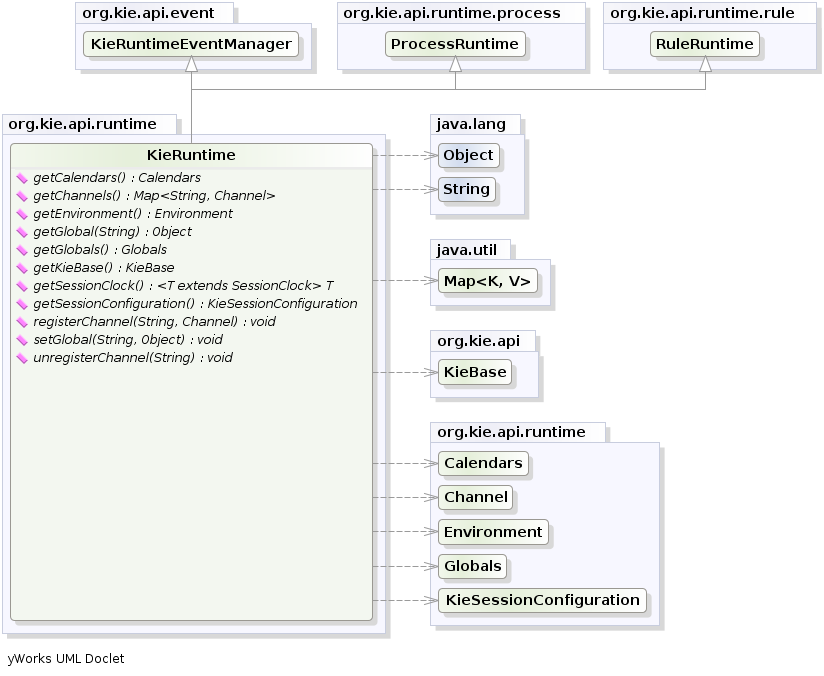
Globals
Globals are named objects that are made visible to the Drools rule engine, but in a way that is fundamentally different from the one for facts: changes in the object backing a global do not trigger reevaluation of rules. Still, globals are useful for providing static information, as an object offering services that are used in the RHS of a rule, or as a means to return objects from the Drools rule engine. When you use a global on the LHS of a rule, make sure it is immutable, or, at least, don’t expect changes to have any effect on the behavior of your rules.
A global must be declared in a rules file, and then it needs to be backed up with a Java object.
global java.util.List list
With the KIE base now aware of the global identifier and its type, it is now possible to call ksession.setGlobal()
with the global’s name and an object, for any session, to associate the object with the global.
Failure to declare the global type and identifier in DRL code will result in an exception being thrown from this call.
List<String> list = new ArrayList<>();
ksession.setGlobal("list", list);
Make sure to set any global before it is used in the evaluation of a rule.
Failure to do so results in a NullPointerException
.
Event Model
The event package provides means to be notified of Drools rule engine events, including rules firing, objects being asserted, etc. This allows separation of logging and auditing activities from the main part of your application (and the rules).
The KieRuntimeEventManager
interface is implemented by the KieRuntime
which provides two interfaces, RuleRuntimeEventManager
and ProcessEventManager
.
We will only cover the RuleRuntimeEventManager
here.
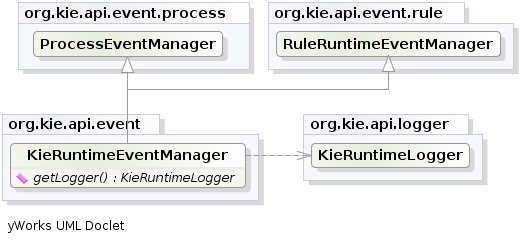
The RuleRuntimeEventManager
allows for listeners to be added and removed, so that events for the working memory and the agenda can be listened to.
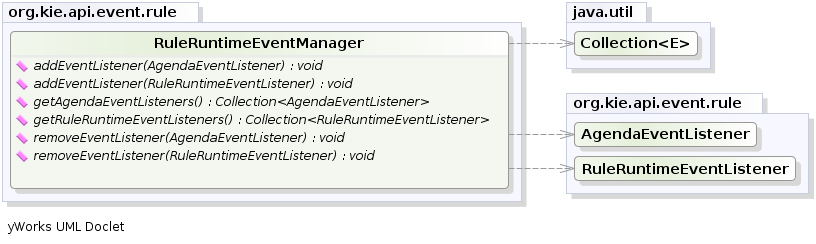
The following code snippet shows how a simple agenda listener is declared and attached to a session. It will print matches after they have fired.
ksession.addEventListener( new DefaultAgendaEventListener() {
public void afterMatchFired(AfterMatchFiredEvent event) {
super.afterMatchFired( event );
System.out.println( event );
}
});
Drools also provides DebugRuleRuntimeEventListener
and DebugAgendaEventListener
which implement each method with a debug print statement.
To print all Working Memory events, you add a listener like this:
ksession.addEventListener( new DebugRuleRuntimeEventListener() );
All emitted events implement the KieRuntimeEvent
interface which can be used to retrieve the actual KnowlegeRuntime
the event originated from.
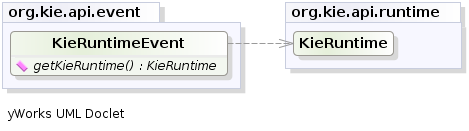
The events currently supported are:
-
MatchCreatedEvent
-
MatchCancelledEvent
-
BeforeMatchFiredEvent
-
AfterMatchFiredEvent
-
AgendaGroupPushedEvent
-
AgendaGroupPoppedEvent
-
ObjectInsertEvent
-
ObjectDeletedEvent
-
ObjectUpdatedEvent
-
ProcessCompletedEvent
-
ProcessNodeLeftEvent
-
ProcessNodeTriggeredEvent
-
ProcessStartEvent
KieRuntimeLogger
The KieRuntimeLogger uses the comprehensive event system in Drools to create an audit log that can be used to log the execution of an application for later inspection, using tools such as the Eclipse audit viewer.
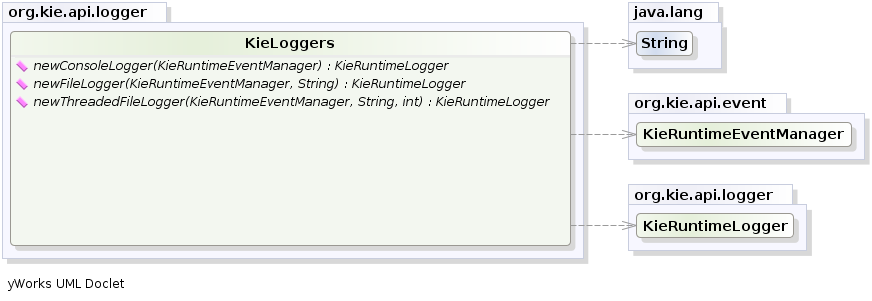
KieRuntimeLogger logger =
KieServices.Factory.get().getLoggers().newFileLogger(ksession, "logdir/mylogfile");
...
logger.close();
Commands and the CommandExecutor
KIE has the concept of stateful or stateless sessions. Stateful sessions have already been covered, which use the standard KieRuntime, and can be worked with iteratively over time. Stateless is a one-off execution of a KieRuntime with a provided data set. It may return some results, with the session being disposed at the end, prohibiting further iterative interactions. You can think of stateless as treating an engine like a function call with optional return results.
The foundation for this is the CommandExecutor
interface, which both the stateful and stateless interfaces extend.
This returns an ExecutionResults
:
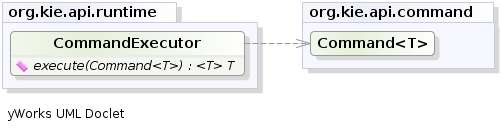
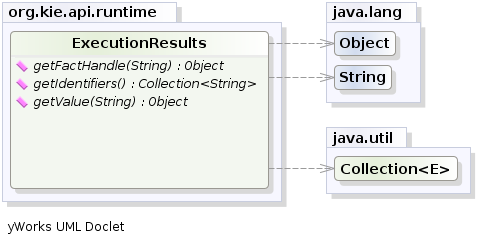
The CommandExecutor
allows for commands to be executed on those sessions, the only difference being that the StatelessKieSession executes fireAllRules()
at the end before disposing the session.
The commands can be created using the CommandExecutor
.The Javadocs provide the full list of the allowed commands using the CommandExecutor
.
setGlobal and getGlobal are two commands relevant to both Drools and jBPM.
Set Global calls setGlobal underneath.
The optional boolean indicates whether the command should return the global’s value as part of the ExecutionResults
.
If true it uses the same name as the global name.
A String can be used instead of the boolean, if an alternative name is desired.
StatelessKieSession ksession = kbase.newStatelessKieSession();
ExecutionResults bresults =
ksession.execute( CommandFactory.newSetGlobal( "stilton", new Cheese( "stilton" ), true);
Cheese stilton = bresults.getValue( "stilton" );
Allows an existing global to be returned. The second optional String argument allows for an alternative return name.
StatelessKieSession ksession = kbase.newStatelessKieSession();
ExecutionResults bresults =
ksession.execute( CommandFactory.getGlobal( "stilton" );
Cheese stilton = bresults.getValue( "stilton" );
All the previous examples execute single commands.
The BatchExecution
represents a composite command, created from a list of commands.
It will iterate over the list and execute each command in turn.
This means you can insert some objects, start a process, call fireAllRules and execute a query, all in a single execute(…)
call, which is quite powerful.
The StatelessKieSession will execute fireAllRules()
automatically at the end.
However the keen-eyed reader probably has already noticed the FireAllRules
command and wondered how that works with a StatelessKieSession.
The FireAllRules
command is allowed, and using it will disable the automatic execution at the end; think of using it as a sort of manual override function.
Any command, in the batch, that has an out identifier set will add its results to the returned ExecutionResults
instance.
Let’s look at a simple example to see how this works.
The example presented includes command from the Drools and jBPM, for the sake of illustration.
They are covered in more detail in the Drool and jBPM specific sections.
StatelessKieSession ksession = kbase.newStatelessKieSession();
List cmds = new ArrayList();
cmds.add( CommandFactory.newInsertObject( new Cheese( "stilton", 1), "stilton") );
cmds.add( CommandFactory.newStartProcess( "process cheeses" ) );
cmds.add( CommandFactory.newQuery( "cheeses" ) );
ExecutionResults bresults = ksession.execute( CommandFactory.newBatchExecution( cmds ) );
Cheese stilton = ( Cheese ) bresults.getValue( "stilton" );
QueryResults qresults = ( QueryResults ) bresults.getValue( "cheeses" );
In the previous example multiple commands are executed, two of which populate the ExecutionResults
.
The query command defaults to use the same identifier as the query name, but it can also be mapped to a different identifier.
StatelessKieSession
The StatelessKieSession
wraps the KieSession
, instead of extending it.
Its main focus is on the decision service type scenarios.
It avoids the need to call dispose()
.
Stateless sessions do not support iterative insertions and the method call fireAllRules()
from Java code; the act of calling execute()
is a single-shot method that will internally instantiate a KieSession
, add all the user data and execute user commands, call fireAllRules()
, and then call dispose()
.
While the main way to work with this class is via the BatchExecution
(a subinterface of Command
) as supported by the CommandExecutor
interface, two convenience methods are provided for when simple object insertion is all that’s required.
The CommandExecutor
and BatchExecution
are talked about in detail in their own section.
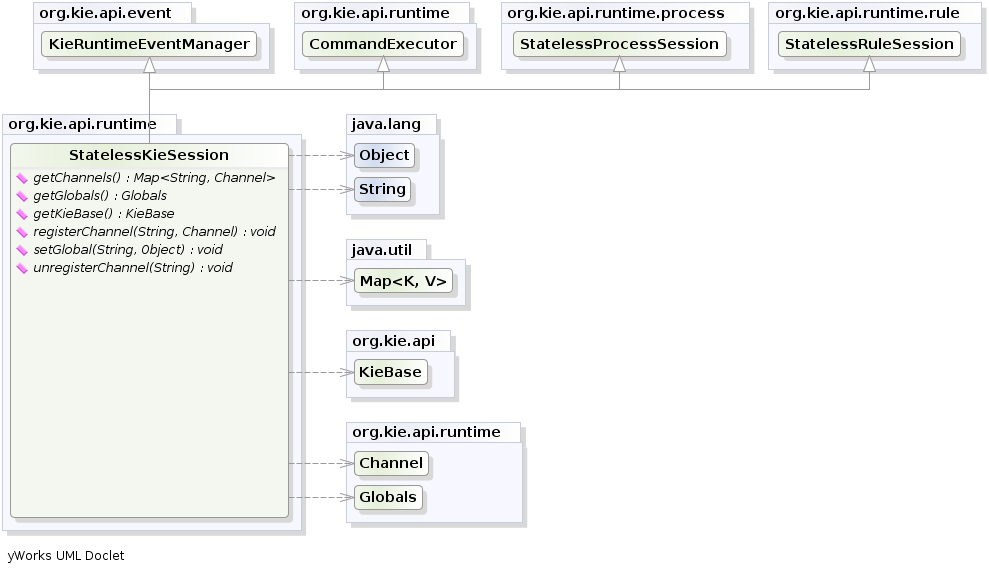
Our simple example shows a stateless session executing a given collection of Java objects using the convenience API. It will iterate the collection, inserting each element in turn.
StatelessKieSession ksession = kbase.newStatelessKieSession();
ksession.execute( collection );
If this was done as a single Command it would be as follows:
ksession.execute( CommandFactory.newInsertElements( collection ) );
If you wanted to insert the collection itself, and the collection’s individual elements, then CommandFactory.newInsert(collection)
would do the job.
Methods of the CommandFactory
create the supported commands, all of which can be marshalled using XStream and the BatchExecutionHelper
. BatchExecutionHelper
provides details on the XML format as well as how to use Drools Pipeline to automate the marshalling of BatchExecution
and ExecutionResults
.
StatelessKieSession
supports globals, scoped in a number of ways.
We cover the non-command way first, as commands are scoped to a specific execution call.
Globals can be resolved in three ways.
-
The StatelessKieSession method
getGlobals()
returns a Globals instance which provides access to the session’s globals. These are used for all execution calls. Exercise caution regarding mutable globals because execution calls can be executing simultaneously in different threads.Example 26. Session scoped globalStatelessKieSession ksession = kbase.newStatelessKieSession(); // Set a global hbnSession, that can be used for DB interactions in the rules. ksession.setGlobal( "hbnSession", hibernateSession ); // Execute while being able to resolve the "hbnSession" identifier. ksession.execute( collection );
-
Using a delegate is another way of global resolution. Assigning a value to a global (with
setGlobal(String, Object)
) results in the value being stored in an internal collection mapping identifiers to values. Identifiers in this internal collection will have priority over any supplied delegate. Only if an identifier cannot be found in this internal collection, the delegate global (if any) will be used. -
The third way of resolving globals is to have execution scoped globals. Here, a
Command
to set a global is passed to theCommandExecutor
.
The CommandExecutor
interface also offers the ability to export data via "out" parameters.
Inserted facts, globals and query results can all be returned.
// Set up a list of commands
List cmds = new ArrayList();
cmds.add( CommandFactory.newSetGlobal( "list1", new ArrayList(), true ) );
cmds.add( CommandFactory.newInsert( new Person( "jon", 102 ), "person" ) );
cmds.add( CommandFactory.newQuery( "Get People", "getPeople" ) );
// Execute the list
ExecutionResults results =
ksession.execute( CommandFactory.newBatchExecution( cmds ) );
// Retrieve the ArrayList
results.getValue( "list1" );
// Retrieve the inserted Person fact
results.getValue( "person" );
// Retrieve the query as a QueryResults instance.
results.getValue( "Get People" );
Marshalling
The KieMarshallers
are used to marshal and unmarshal KieSessions.
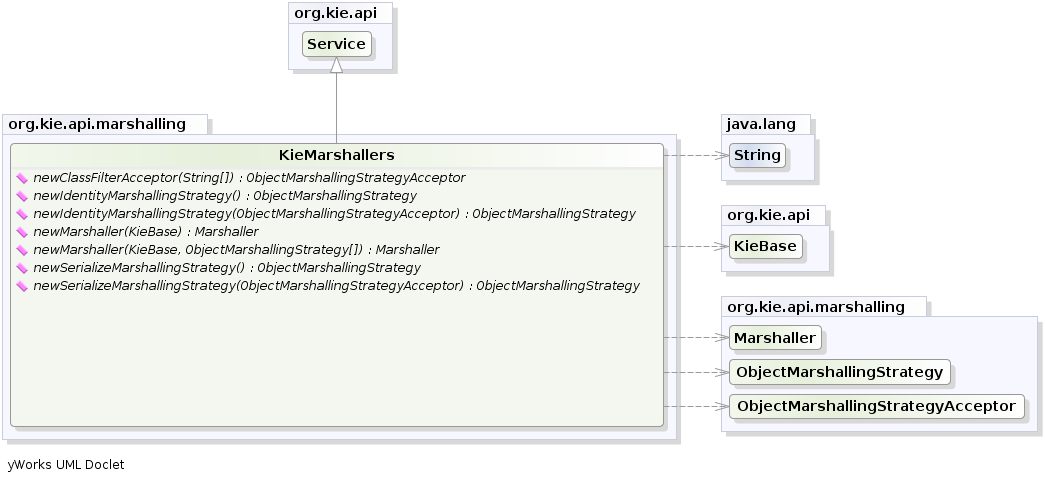
An instance of the KieMarshallers
can be retrieved from the KieServices
.
A simple example is shown below:
// ksession is the KieSession
// kbase is the KieBase
ByteArrayOutputStream baos = new ByteArrayOutputStream();
Marshaller marshaller = KieServices.Factory.get().getMarshallers().newMarshaller( kbase );
marshaller.marshall( baos, ksession );
baos.close();
However, with marshalling, you will need more flexibility when dealing with referenced user data.
To achieve this use the ObjectMarshallingStrategy
interface.
Two implementations are provided, but users can implement their own.
The two supplied strategies are IdentityMarshallingStrategy
and SerializeMarshallingStrategy
. SerializeMarshallingStrategy
is the default, as shown in the example above, and it just calls the Serializable
or Externalizable
methods on a user instance. IdentityMarshallingStrategy
creates an integer id for each user object and stores them in a Map, while the id is written to the stream.
When unmarshalling it accesses the IdentityMarshallingStrategy
map to retrieve the instance.
This means that if you use the IdentityMarshallingStrategy
, it is stateful for the life of the Marshaller instance and will create ids and keep references to all objects that it attempts to marshal.
Below is the code to use an Identity Marshalling Strategy.
ByteArrayOutputStream baos = new ByteArrayOutputStream();
KieMarshallers kMarshallers = KieServices.Factory.get().getMarshallers()
ObjectMarshallingStrategy oms = kMarshallers.newIdentityMarshallingStrategy()
Marshaller marshaller =
kMarshallers.newMarshaller( kbase, new ObjectMarshallingStrategy[]{ oms } );
marshaller.marshall( baos, ksession );
baos.close();
In most cases, a single strategy is insufficient.
For added flexibility, the ObjectMarshallingStrategyAcceptor
interface can be used.
This Marshaller has a chain of strategies, and while reading or writing a user object it iterates the strategies asking if they accept responsibility for marshalling the user object.
One of the provided implementations is ClassFilterAcceptor
.
This allows strings and wild cards to be used to match class names.
The default is ".", so in the previous example the Identity Marshalling Strategy is used which has a default "." acceptor.
Assuming that we want to serialize all classes except for one given package, where we will use identity lookup, we could do the following:
ByteArrayOutputStream baos = new ByteArrayOutputStream();
KieMarshallers kMarshallers = KieServices.Factory.get().getMarshallers()
ObjectMarshallingStrategyAcceptor identityAcceptor =
kMarshallers.newClassFilterAcceptor( new String[] { "org.domain.pkg1.*" } );
ObjectMarshallingStrategy identityStrategy =
kMarshallers.newIdentityMarshallingStrategy( identityAcceptor );
ObjectMarshallingStrategy sms = kMarshallers.newSerializeMarshallingStrategy();
Marshaller marshaller =
kMarshallers.newMarshaller( kbase,
new ObjectMarshallingStrategy[]{ identityStrategy, sms } );
marshaller.marshall( baos, ksession );
baos.close();
Note that the acceptance checking order is in the natural order of the supplied elements.
Persistence and Transactions
Longterm out of the box persistence with Java Persistence API (JPA) is possible with Drools. It is necessary to have some implementation of the Java Transaction API (JTA) installed. For development purposes the Bitronix Transaction Manager is suggested, as it’s simple to set up and works embedded, but for production use JBoss Transactions is recommended.
KieServices kieServices = KieServices.Factory.get();
Environment env = kieServices.newEnvironment();
env.set( EnvironmentName.ENTITY_MANAGER_FACTORY,
Persistence.createEntityManagerFactory( "emf-name" ) );
env.set( EnvironmentName.TRANSACTION_MANAGER,
TransactionManagerServices.getTransactionManager() );
// KieSessionConfiguration may be null, and a default will be used
KieSession ksession =
kieServices.getStoreServices().newKieSession( kbase, null, env );
int sessionId = ksession.getId();
UserTransaction ut =
(UserTransaction) new InitialContext().lookup( "java:comp/UserTransaction" );
ut.begin();
ksession.insert( data1 );
ksession.insert( data2 );
ksession.startProcess( "process1" );
ut.commit();
To use a JPA, the Environment must be set with both the EntityManagerFactory
and the TransactionManager
.
If rollback occurs the ksession state is also rolled back, hence it is possible to continue to use it after a rollback.
To load a previously persisted KieSession you’ll need the id, as shown below:
KieSession ksession =
kieServices.getStoreServices().loadKieSession( sessionId, kbase, null, env );
To enable persistence several classes must be added to your persistence.xml, as in the example below:
<persistence-unit name="org.drools.persistence.jpa" transaction-type="JTA">
<provider>org.hibernate.ejb.HibernatePersistence</provider>
<jta-data-source>jdbc/BitronixJTADataSource</jta-data-source>
<class>org.drools.persistence.info.SessionInfo</class>
<class>org.drools.persistence.info.WorkItemInfo</class>
<properties>
<property name="hibernate.dialect" value="org.hibernate.dialect.H2Dialect"/>
<property name="hibernate.max_fetch_depth" value="3"/>
<property name="hibernate.hbm2ddl.auto" value="update" />
<property name="hibernate.show_sql" value="true" />
<property name="hibernate.transaction.manager_lookup_class"
value="org.hibernate.transaction.BTMTransactionManagerLookup" />
</properties>
</persistence-unit>
The jdbc JTA data source would have to be configured first. Bitronix provides a number of ways of doing this, and its documentation should be consulted for details. For a quick start, here is the programmatic approach:
PoolingDataSource fs = new PoolingDataSource();
fs.setUniqueName( "jdbc/BitronixJTADataSource" );
fs.setClassName( "org.h2.jdbcx.JdbcDataSource" );
fs.setMaxPoolSize( 3 );
fs.setAllowLocalTransactions( true );
fs.getDriverProperties().put( "user", "sa" );
fs.getDriverProperties().put( "password", "sasa" );
fs.getDriverProperties().put( "URL", "jdbc:h2:mem:mydb" );
fs.init();
Bitronix also provides a simple embedded JNDI service, ideal for testing. To use it, add a jndi.properties file to your META-INF folder and add the following line to it:
java.naming.factory.initial=bitronix.tm.jndi.BitronixInitialContextFactory
Build, Deploy and Utilize Examples
The best way to learn the new build system is by example. The source project "drools-examples-api" contains a number of examples, and can be found at GitHub:
Each example is described below, the order starts with the simplest (most of the options are defaulted) and working its way up to more complex use cases.
The Deploy use cases shown below all involve mvn install
.
Remote deployment of JARs in Maven is well covered in Maven literature.
Utilize refers to the initial act of loading the resources and providing access to the KIE runtimes.
Whereas Run refers to the act of interacting with those runtimes.
Default KieSession
-
Project: default-kesession.
-
Summary: Empty kmodule.xml KieModule on the classpath that includes all resources in a single default KieBase. The example shows the retrieval of the default KieSession from the classpath.
An empty kmodule.xml will produce a single KieBase that includes all files found under resources path, be it DRL, BPMN2, XLS etc. That single KieBase is the default and also includes a single default KieSession. Default means they can be created without knowing their names.
<kmodule xmlns="http://www.drools.org/xsd/kmodule"> </kmodule>
mvn install
ks.getKieClasspathContainer() returns the KieContainer that contains the KieBases deployed onto the environment classpath. kContainer.newKieSession() creates the default KieSession. Notice that you no longer need to look up the KieBase, in order to create the KieSession. The KieSession knows which KieBase it’s associated with, and use that, which in this case is the default KieBase.
KieServices ks = KieServices.Factory.get();
KieContainer kContainer = ks.getKieClasspathContainer();
KieSession kSession = kContainer.newKieSession();
kSession.setGlobal("out", out);
kSession.insert(new Message("Dave", "Hello, HAL. Do you read me, HAL?"));
kSession.fireAllRules();
Named KieSession
-
Project: named-kiesession.
-
Summary: kmodule.xml that has one named KieBase and one named KieSession. The examples shows the retrieval of the named KieSession from the classpath.
kmodule.xml will produce a single named KieBase, 'kbase1' that includes all files found under resources path, be it DRL, BPMN2, XLS etc. KieSession 'ksession1' is associated with that KieBase and can be created by name.
<kmodule xmlns="http://www.drools.org/xsd/kmodule">
<kbase name="kbase1">
<ksession name="ksession1"/>
</kbase>
</kmodule>
mvn install
ks.getKieClasspathContainer() returns the KieContainer that contains the KieBases deployed onto the environment classpath. This time the KieSession uses the name 'ksession1'. You do not need to lookup the KieBase first, as it knows which KieBase 'ksession1' is assocaited with.
KieServices ks = KieServices.Factory.get();
KieContainer kContainer = ks.getKieClasspathContainer();
KieSession kSession = kContainer.newKieSession("ksession1");
kSession.setGlobal("out", out);
kSession.insert(new Message("Dave", "Hello, HAL. Do you read me, HAL?"));
kSession.fireAllRules();
KieBase Inheritance
-
Project: kiebase-inclusion.
-
Summary: 'kmodule.xml' demonstrates that one KieBase can include the resources from another KieBase, from another KieModule. In this case it inherits the named KieBase from the 'name-kiesession' example. The included KieBase can be from the current KieModule or any other KieModule that is in the pom.xml dependency list.
kmodule.xml will produce a single named KieBase, 'kbase2' that includes all files found under resources path, be it DRL, BPMN2, XLS etc. Further it will include all the resources found from the KieBase 'kbase1', due to the use of the 'includes' attribute. KieSession 'ksession2' is associated with that KieBase and can be created by name.
<kbase name="kbase2" includes="kbase1">
<ksession name="ksession2"/>
</kbase>
This example requires that the previous example, 'named-kiesession', is built and installed to the local Maven repository first. Once installed it can be included as a dependency, using the standard Maven <dependencies> element.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.drools</groupId>
<artifactId>drools-examples-api</artifactId>
<version>6.0.0/version>
</parent>
<artifactId>kiebase-inclusion</artifactId>
<name>Drools API examples - KieBase Inclusion</name>
<dependencies>
<dependency>
<groupId>org.drools</groupId>
<artifactId>drools-compiler</artifactId>
</dependency>
<dependency>
<groupId>org.drools</groupId>
<artifactId>named-kiesession</artifactId>
<version>6.0.0</version>
</dependency>
</dependencies>
</project>
Once 'named-kiesession' is built and installed this example can be built and installed as normal. Again the act of installing, will force the unit tests to run, demonstrating the use case.
mvn install
ks.getKieClasspathContainer() returns the KieContainer that contains the KieBases deployed onto the environment classpath. This time the KieSession uses the name 'ksession2'. You do not need to lookup the KieBase first, as it knows which KieBase 'ksession1' is assocaited with. Notice two rules fire this time, showing that KieBase 'kbase2' has included the resources from the dependency KieBase 'kbase1'.
KieServices ks = KieServices.Factory.get();
KieContainer kContainer = ks.getKieClasspathContainer();
KieSession kSession = kContainer.newKieSession("ksession2");
kSession.setGlobal("out", out);
kSession.insert(new Message("Dave", "Hello, HAL. Do you read me, HAL?"));
kSession.fireAllRules();
kSession.insert(new Message("Dave", "Open the pod bay doors, HAL."));
kSession.fireAllRules();
Multiple KieBases
-
Project: 'multiple-kbases.
-
Summary: Demonstrates that the 'kmodule.xml' can contain any number of KieBase or KieSession declarations. Introduces the 'packages' attribute to select the folders for the resources to be included in the KieBase.
kmodule.xml produces 6 different named KieBases. 'kbase1' includes all resources from the KieModule. The other KieBases include resources from other selected folders, via the 'packages' attribute. Note the use of wildcard '*', to select this package and all packages below it.
<kmodule xmlns="http://www.drools.org/xsd/kmodule">
<kbase name="kbase1">
<ksession name="ksession1"/>
</kbase>
<kbase name="kbase2" packages="org.some.pkg">
<ksession name="ksession2"/>
</kbase>
<kbase name="kbase3" includes="kbase2" packages="org.some.pkg2">
<ksession name="ksession3"/>
</kbase>
<kbase name="kbase4" packages="org.some.pkg, org.other.pkg">
<ksession name="ksession4"/>
</kbase>
<kbase name="kbase5" packages="org.*">
<ksession name="ksession5"/>
</kbase>
<kbase name="kbase6" packages="org.some.*">
<ksession name="ksession6"/>
</kbase>
</kmodule>
mvn install
Only part of the example is included below, as there is a test method per KieSession, but each one is a repetition of the other, with different list expectations.
@Test
public void testSimpleKieBase() {
List<Integer> list = useKieSession("ksession1");
// no packages imported means import everything
assertEquals(4, list.size());
assertTrue( list.containsAll( asList(0, 1, 2, 3) ) );
}
//.. other tests for ksession2 to ksession6 here
private List<Integer> useKieSession(String name) {
KieServices ks = KieServices.Factory.get();
KieContainer kContainer = ks.getKieClasspathContainer();
KieSession kSession = kContainer.newKieSession(name);
List<Integer> list = new ArrayList<Integer>();
kSession.setGlobal("list", list);
kSession.insert(1);
kSession.fireAllRules();
return list;
}
KieContainer from KieRepository
-
Project: kcontainer-from-repository
-
Summary: The project does not contain a kmodule.xml, nor does the pom.xml have any dependencies for other KieModules. Instead the Java code demonstrates the loading of a dynamic KieModule from a Maven repository.
The pom.xml must include kie-ci as a dependency, to ensure Maven is available at runtime. As this uses Maven under the hood you can also use the standard Maven settings.xml file.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.drools</groupId>
<artifactId>drools-examples-api</artifactId>
<version>6.0.0</version>
</parent>
<artifactId>kiecontainer-from-kierepo</artifactId>
<name>Drools API examples - KieContainer from KieRepo</name>
<dependencies>
<dependency>
<groupId>org.kie</groupId>
<artifactId>kie-ci</artifactId>
</dependency>
</dependencies>
</project>
mvn install
In the previous examples the classpath KieContainer used. This example creates a dynamic KieContainer as specified by the ReleaseId. The ReleaseId uses Maven conventions for group id, artifact id and version. It also obeys LATEST and SNAPSHOT for versions.
KieServices ks = KieServices.Factory.get();
// Install example1 in the local Maven repo before to do this
KieContainer kContainer = ks.newKieContainer(ks.newReleaseId("org.drools", "named-kiesession", "6.0.0-SNAPSHOT"));
KieSession kSession = kContainer.newKieSession("ksession1");
kSession.setGlobal("out", out);
Object msg1 = createMessage(kContainer, "Dave", "Hello, HAL. Do you read me, HAL?");
kSession.insert(msg1);
kSession.fireAllRules();
Default KieSession from File
-
Project: default-kiesession-from-file
-
Summary: Dynamic KieModules can also be loaded from any Resource location. The loaded KieModule provides default KieBase and KieSession definitions.
No kmodue.xml file exists. The project 'default-kiesession' must be built first, so that the resulting JAR, in the target folder, can be referenced as a File.
mvn install
Any KieModule can be loaded from a Resource location and added to the KieRepository. Once deployed in the KieRepository it can be resolved via its ReleaseId. Note neither Maven or kie-ci are needed here. It will not set up a transitive dependency parent classloader.
KieServices ks = KieServices.Factory.get();
KieRepository kr = ks.getRepository();
KieModule kModule = kr.addKieModule(ks.getResources().newFileSystemResource(getFile("default-kiesession")));
KieContainer kContainer = ks.newKieContainer(kModule.getReleaseId());
KieSession kSession = kContainer.newKieSession();
kSession.setGlobal("out", out);
Object msg1 = createMessage(kContainer, "Dave", "Hello, HAL. Do you read me, HAL?");
kSession.insert(msg1);
kSession.fireAllRules();
Named KieSession from File
-
Project: named-kiesession-from-file
-
Summary: Dynamic KieModules can also be loaded from any Resource location. The loaded KieModule provides named KieBase and KieSession definitions.
No kmodue.xml file exists. The project 'named-kiesession' must be built first, so that the resulting JAR, in the target folder, can be referenced as a File.
mvn install
Any KieModule can be loaded from a Resource location and added to the KieRepository. Once in the KieRepository it can be resolved via its ReleaseId. Note neither Maven or kie-ci are needed here. It will not setup a transitive dependency parent classloader.
KieServices ks = KieServices.Factory.get();
KieRepository kr = ks.getRepository();
KieModule kModule = kr.addKieModule(ks.getResources().newFileSystemResource(getFile("named-kiesession")));
KieContainer kContainer = ks.newKieContainer(kModule.getReleaseId());
KieSession kSession = kContainer.newKieSession("ksession1");
kSession.setGlobal("out", out);
Object msg1 = createMessage(kContainer, "Dave", "Hello, HAL. Do you read me, HAL?");
kSession.insert(msg1);
kSession.fireAllRules();
KieModule with Dependent KieModule
-
Project: kie-module-form-multiple-files
-
Summary: Programmatically provide the list of dependant KieModules, without using Maven to resolve anything.
No kmodue.xml file exists. The projects 'named-kiesession' and 'kiebase-include' must be built first, so that the resulting JARs, in the target folders, can be referenced as Files.
mvn install
Creates two resources. One is for the main KieModule 'exRes1' the other is for the dependency 'exRes2'. Even though kie-ci is not present and thus Maven is not available to resolve the dependencies, this shows how you can manually specify the dependent KieModules, for the vararg.
KieServices ks = KieServices.Factory.get();
KieRepository kr = ks.getRepository();
Resource ex1Res = ks.getResources().newFileSystemResource(getFile("kiebase-inclusion"));
Resource ex2Res = ks.getResources().newFileSystemResource(getFile("named-kiesession"));
KieModule kModule = kr.addKieModule(ex1Res, ex2Res);
KieContainer kContainer = ks.newKieContainer(kModule.getReleaseId());
KieSession kSession = kContainer.newKieSession("ksession2");
kSession.setGlobal("out", out);
Object msg1 = createMessage(kContainer, "Dave", "Hello, HAL. Do you read me, HAL?");
kSession.insert(msg1);
kSession.fireAllRules();
Object msg2 = createMessage(kContainer, "Dave", "Open the pod bay doors, HAL.");
kSession.insert(msg2);
kSession.fireAllRules();
Programmatically build a Simple KieModule with Defaults
-
Project: kiemoduelmodel-example
-
Summary: Programmatically build a KieModule from just a single file. The POM and models are all defaulted. This is the quickest out of the box approach, but should not be added to a Maven repository.
mvn install
This programmatically builds a KieModule. It populates the model that represents the ReleaseId and kmodule.xml, and it adds the relevant resources. A pom.xml is generated from the ReleaseId.
KieServices ks = KieServices.Factory.get();
KieRepository kr = ks.getRepository();
KieFileSystem kfs = ks.newKieFileSystem();
kfs.write("src/main/resources/org/kie/example5/HAL5.drl", getRule());
KieBuilder kb = ks.newKieBuilder(kfs);
kb.buildAll(); // kieModule is automatically deployed to KieRepository if successfully built.
if (kb.getResults().hasMessages(Level.ERROR)) {
throw new RuntimeException("Build Errors:\n" + kb.getResults().toString());
}
KieContainer kContainer = ks.newKieContainer(kr.getDefaultReleaseId());
KieSession kSession = kContainer.newKieSession();
kSession.setGlobal("out", out);
kSession.insert(new Message("Dave", "Hello, HAL. Do you read me, HAL?"));
kSession.fireAllRules();
Programmatically build a KieModule using Meta Models
-
Project: kiemoduelmodel-example
-
Summary: Programmatically build a KieModule, by creating its kmodule.xml meta model resources.
mvn install
This programmatically builds a KieModule. It populates the model that represents the ReleaseId and kmodule.xml, as well as add the relevant resources. A pom.xml is generated from the ReleaseId.
KieServices ks = KieServices.Factory.get();
KieFileSystem kfs = ks.newKieFileSystem();
Resource ex1Res = ks.getResources().newFileSystemResource(getFile("named-kiesession"));
Resource ex2Res = ks.getResources().newFileSystemResource(getFile("kiebase-inclusion"));
ReleaseId rid = ks.newReleaseId("org.drools", "kiemodulemodel-example", "6.0.0-SNAPSHOT");
kfs.generateAndWritePomXML(rid);
KieModuleModel kModuleModel = ks.newKieModuleModel();
kModuleModel.newKieBaseModel("kiemodulemodel")
.addInclude("kiebase1")
.addInclude("kiebase2")
.newKieSessionModel("ksession6");
kfs.writeKModuleXML(kModuleModel.toXML());
kfs.write("src/main/resources/kiemodulemodel/HAL6.drl", getRule());
KieBuilder kb = ks.newKieBuilder(kfs);
kb.setDependencies(ex1Res, ex2Res);
kb.buildAll(); // kieModule is automatically deployed to KieRepository if successfully built.
if (kb.getResults().hasMessages(Level.ERROR)) {
throw new RuntimeException("Build Errors:\n" + kb.getResults().toString());
}
KieContainer kContainer = ks.newKieContainer(rid);
KieSession kSession = kContainer.newKieSession("ksession6");
kSession.setGlobal("out", out);
Object msg1 = createMessage(kContainer, "Dave", "Hello, HAL. Do you read me, HAL?");
kSession.insert(msg1);
kSession.fireAllRules();
Object msg2 = createMessage(kContainer, "Dave", "Open the pod bay doors, HAL.");
kSession.insert(msg2);
kSession.fireAllRules();
Object msg3 = createMessage(kContainer, "Dave", "What's the problem?");
kSession.insert(msg3);
kSession.fireAllRules();
Executable rule models
Rule assets in Drools are built from executable rule models by default with the standard kie-maven-plugin
plugin. Executable rule models are embedded models that provide a Java-based representation of a rule set for execution at build time. The executable model is a more efficient alternative to the standard asset packaging in previous versions of Drools and enables KIE containers and KIE bases to be created more quickly, especially when you have large lists of DRL (Drools Rule Language) files and other Drools assets.
If you do not use the kie-maven-plugin
plugin or if the required drools-model-compiler
dependency is missing from your project, then rule assets are built without executable models. Therefore, to generate the executable model during build time, ensure that the kie-maven-plugin
plugin and drools-model-compiler
dependency are added in your project pom.xml
file.
Starting from Drools 8.33, if your project has |
Executable rule models provide the following specific advantages for your projects:
-
Compile time: Traditionally, a packaged Drools project (KJAR) contains a list of DRL files and other Drools artifacts that define the rule base together with some pre-generated classes implementing the constraints and the consequences. Those DRL files must be parsed and compiled when the KJAR is downloaded from the Maven repository and installed in a KIE container. This process can be slow, especially for large rule sets. With an executable model, you can package within the project KJAR the Java classes that implement the executable model of the project rule base and re-create the KIE container and its KIE bases out of it in a much faster way. In Maven projects, you use the
kie-maven-plugin
plugin to automatically generate the executable model sources from the DRL files during the compilation process. -
Run time: In an executable model, all constraints are defined as Java lambda expressions. The same lambda expressions are also used for constraints evaluation, so you no longer need to use
mvel
expressions for interpreted evaluation nor the just-in-time (JIT) process to transform themvel
-based constraints into bytecode. This creates a quicker and more efficient run time. -
Development time: An executable model enables you to develop and experiment with new features of the Drools rule engine without needing to encode elements directly in the DRL format or modify the DRL parser to support them.
For query definitions in executable rule models, you can use up to 10 arguments only. For variables within rule consequences in executable rule models, you can use up to 24 bound variables only (including the built-in
|
Modifying or disabling executable rule models in a Drools project
Rule assets in Drools are built from executable rule models by default with the standard kie-maven-plugin
plugin. The executable model is a more efficient alternative to the standard asset packaging in previous versions of Drools. However, if needed, you can modify or disable executable rule models to build a Drools project as a DRL-based KJAR instead of the default model-based KJAR.
Build your Drools project in the usual way, but provide an alternate build option, depending on the type of project:
-
For a Maven project, navigate to your Maven project directory in a command terminal and run the following command:
mvn clean install -DgenerateModel=<VALUE>
Replace
<VALUE>
with one of three values:-
YES_WITHDRL
: (Default) Generates the executable model corresponding to the DRL files in the original project and also adds the DRL files to the generated KJAR for documentation purposes (the KIE base is built from the executable model regardless). -
YES
: Generates the executable model corresponding to the DRL files in the original project and excludes the DRL files from the generated KJAR. -
NO
: Does not generate the executable model.
Example build command to disable the default executable model behavior:
mvn clean install -DgenerateModel=NO
-
-
For a Java application configured programmatically, the executable model is disabled by default. Add rule assets to the KIE virtual file system
KieFileSystem
and useKieBuilder
with one of the followingbuildAll()
methods:-
buildAll()
(Default) orbuildAll(DrlProject.class)
: Does not generate the executable model. -
buildAll(ExecutableModelProject.class)
: Generates the executable model corresponding to the DRL files in the original project.
Example code to enable executable model behavior:
import org.kie.api.KieServices; import org.kie.api.builder.KieFileSystem; import org.kie.api.builder.KieBuilder; KieServices ks = KieServices.Factory.get(); KieFileSystem kfs = ks.newKieFileSystem() kfs.write("src/main/resources/KBase1/ruleSet1.drl", stringContainingAValidDRL) .write("src/main/resources/dtable.xls", kieServices.getResources().newInputStreamResource(dtableFileStream)); KieBuilder kieBuilder = ks.newKieBuilder( kfs ); // Enable executable model kieBuilder.buildAll(ExecutableModelProject.class) assertEquals(0, kieBuilder.getResults().getMessages(Message.Level.ERROR).size());
-
Enabling executable rule models when upgrading to Drools 7.39
Beginning in Drools 7.39, rule assets are built from executable rule models by default with the standard kie-maven-plugin
plugin. The executable model is a more efficient alternative to the standard asset packaging in previous versions of Drools.
When you install Drools 7.39, this default executable model behavior is configured for all new projects that you create going forward. However, if you are upgrading to Drools 7.39 from a previous version of the product and you have not already enabled executable rule models, you must add the required dependency to your existing Drools projects so that your rule assets are built from executable models in Drools 7.39. If you do not use the kie-maven-plugin
plugin or if the required drools-model-compiler
dependency is missing from your project, then rule assets are built without executable models.
For more information about executable rule models, see Executable rule models.
In the pom.xml
file of your Maven project or on the relevant class path of your Java project, add the following dependency to enable rule assets to be built from the default executable model:
<dependency>
<groupId>org.drools</groupId>
<artifactId>drools-model-compiler</artifactId>
<version>${drools.version}</version>
</dependency>
This dependency compiles the executable model into Drools internal data structures so that it can be executed by the Drools rule engine.
The <version>
is the Maven artifact version for Drools currently used in your project (for example, 7.59.0.Final).
Rule Unit API
Previous sections in this chapter explained traditional KIE APIs to use Drools rule engine. However, as introduced in First Rule Project, Rule Unit is a new and recommended style for implementing rules in Drools 8.
A rule unit is an atomic module defining a set of rules and a set of strongly typed data sources through which the facts processed by the rules are inserted. The data sources of 2 kinds: DataStream
and DataStore
which will be described later in this section.
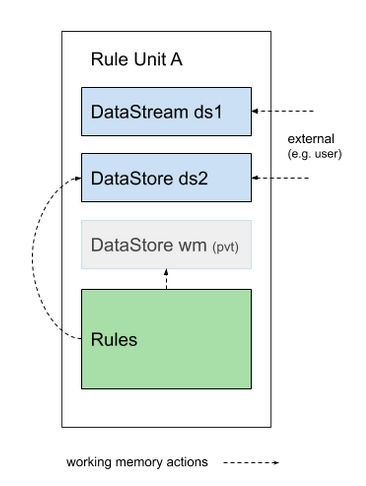
DataSources
can be shared across different units, providing a coordination mechanism between them.
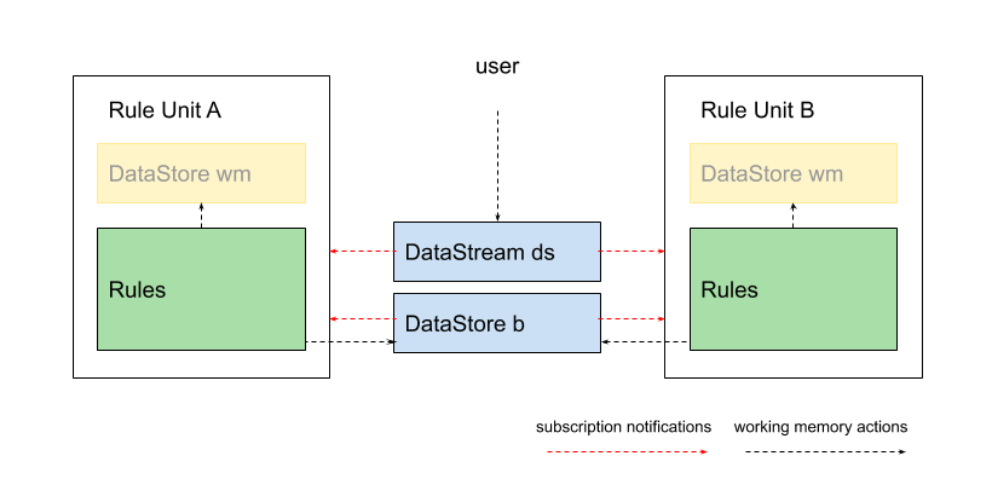
RuleUnitData
RuleUnitData
is an interface to define your Rule Unit. Rule unit implementation would be like this:
public class MeasurementUnit implements RuleUnitData {
private final DataStore<Measurement> measurements;
private final Set<String> controlSet = new HashSet<>();
public MeasurementUnit() {
this(DataSource.createStore());
}
public MeasurementUnit(DataStore<Measurement> measurements) {
this.measurements = measurements;
}
public DataStore<Measurement> getMeasurements() {
return measurements;
}
public Set<String> getControlSet() {
return controlSet;
}
}
In this case, Measurement
is your fact class, so you need to implement it as well.
The name of the rule unit class MeasurementUnit
is linked to DRL rules using the unit
statement.
package org.example;
unit MeasurementUnit;
rule "will execute per each Measurement having ID color"
when
....
Data sources
Data sources are typed sources of data that rule units can subscribe to for updates. You interact with the rule unit through the data sources it exposes.
Drools supports the following types of data sources.
-
DataStream
: An append-only storage option. Use this storage option when you want to publish or share data values. You can use the notationDataSource.createStream()
to return aDataStream<T>
object and use the methodappend(T)
to add more data.Example DataStream data source usageDataStream<Measurement> measurements = DataSource.createStream(); // Append value and notify all subscribers measurements.append(new Measurement("color", "red"));
-
DataStore
: A writable storage option for adding or removing data and then notifying all subscribers that mutable data has been modified. Rules can pattern-match against incoming values and update or remove available values. For users familiar with traditional DRL syntax, this option is equivalent to a typed version of an entry point. In fact, aDataStore<Object>
is equivalent to a traditional-style entry point.Example DataStore data source usageDataStore<Measurement> measurements = DataSource.createStore(); Measurement measurement = new Measurement("color", "red"); // Add value and notify all subscribers DataHandle mHandle = measurements.add(measurement); measure.setValue("blue"); // Notify all subscribers that the value referenced by `mHandle` has changed measurements.update(mHandle, measurement); // Remove value referenced by `mHandle` and notify all subscribers measurements.remove(mHandle);
-
SingletonStore
: A writable storage option for setting or clearing a single element and then notifying all subscribers that the element has been modified. Rules can pattern-match against the value and update or clear available values. For users familiar with traditional DRL syntax, this option is similar to a global but reactive to changes. ASingleton<Object>
is similar to a traditional-style global, except that when used in conjunction with rules, you can pattern-match against it.Example SingletonStore data source definitionSingletonStore<Measurement> measurement = DataSource.createSingleton(); Measurement m1 = new Measurement("color", "red"); // Add value `m1` and notify all subscribers measurement.set(m1); measure.setValue("blue"); // Notify all subscribers that the value has changed measurement.update(); Measurement m2 = new Measurement("color", "green"); // Overwrite contained value with `m2` and notify all subscribers measurement.set(m2); measure2.setValue("black"); // Notify all subscribers that the value has changed measurement.update(); // Clear store and notify all subscribers measurement.clear();
Subscribers to a data source are known as data processors. A data processor implements the DataProcessor<T>
interface. This interface contains callbacks to all the events that a subscribed data source can trigger.
public interface DataProcessor<T> {
default void insert(T object) {
insert(null, object);
}
FactHandle insert(DataHandle handle, T object);
void update(DataHandle handle, T object);
void delete(DataHandle handle);
}
The DataHandle
is an internal reference to an object of a data source. Each callback method might or might not be invoked, depending on whether the corresponding data source implements the capability. For example, a DataStream
source invokes only the insert
callback, whereas a SingletonStore
source invokes the insert
callback on set
and the delete
callback on clear
or before an overwriting set
.
Note that DataProcessor
is a little internal detail. If you instantiate RuleUnitInstance
, EntryPointDataProcessor
is automatically bound to the rule unit’s data sources.
Client code
Finally, you instantiate a RuleUnitInstance
using RuleUnitProvider
to execute the rules.
public void test() {
MeasurementUnit measurementUnit = new MeasurementUnit();
RuleUnitInstance<MeasurementUnit> instance = RuleUnitProvider.get().createRuleUnitInstance(measurementUnit);
try {
measurementUnit.getMeasurements().add(new Measurement("color", "red"));
...
List<Measurement> queryResult = instance.executeQuery("FindColor").stream().map(tuple -> (Measurement) tuple.get("$m")).collect(toList());
...
} finally {
instance.dispose();
}
}
Configurations
You can add configurations by creating RuleUnitInstance
with RuleConfig
.
RuleConfig ruleConfig = RuleUnitProvider.get().newRuleConfig();
ruleConfig.getAgendaEventListeners().add(new MyAgendaEventListener());
ruleConfig.getRuleRuntimeListeners().add(new MyRuleRuntimeEventListener());
ruleConfig.getRuleEventListeners().add(new MyRuleEventListener());
HelloWorldUnit unit = new HelloWorldUnit();
RuleUnitInstance<HelloWorldUnit> unitInstance = RuleUnitProvider.get().createRuleUnitInstance(unit, ruleConfig);
Declare rule units in DRL
Instead of writing a Java class, you can declare rule units directly in DRL. See Rule units in DRL.
Rule Unit DSL
In addition to the standard Rule Unit API, Drools 8 offers an alternative way of writing rules in combination with Rule Unit. You can now define the rules for Rule Units using a dedicated set of Java APIs. Let’s learn with comments on the example.
public class HelloWorldUnit implements RuleUnitDefinition {
private final DataStore<String> strings; // DataStore where you add String fact
private final DataStore<Integer> ints; // DataStore where you add Integer fact
private final List<String> results = new ArrayList<>(); // Store results. In traditional DRL, it is called `global`
// omitting constructors and getters
// ...
@Override
public void defineRules(RulesFactory rulesFactory) {
// /strings[ this == "Hello World" ]
rulesFactory.rule()
.on(strings)
.filter(EQUAL, "Hello World") // when no extractor is provided "this" is implicit
.execute(results, r -> r.add("it worked!")); // the consequence can ignore the matched facts
// /strings[ length > 5 ]
rulesFactory.rule()
.on(strings) // since the datasource has been already initialized its class can be inferred without the need of explicitly passing it
.filter(s -> s.length(), GREATER_THAN, 5) // when no property name is provided it's impossible to generate indexes and property reactivity
.execute(results, (r, s) -> r.add("it also worked with " + s.toUpperCase())); // this consequence also uses the matched fact
// /strings[ length < 5 ]
rulesFactory.rule("MyRule") // it is possible to optionally set a name for the rule
.on(strings)
.filter("length", s -> s.length(), LESS_THAN, 5) // providing the name of the property used in the constraint allows index and property reactivity generation
.execute(results, r -> r.add("this shouldn't fire"));
// $s: /strings[ length > 5 ]
// /ints[ this > 5, this == $s.length ]
rulesFactory.rule()
.on(strings)
.filter("length", s -> s.length() > 5) // it is also possible to use a plain lambda predicate, but in this case no index can be generated
.join(
rule -> rule.on(ints) // creates a new pattern ...
.filter(GREATER_THAN, 5) // ... add an alpha filter to it
) // ... and join it with the former one
.filter(EQUAL, String::length) // this filter is applied to the result of the join, so it is a beta constraint
.execute(results, (r, s, i) -> r.add("String '" + s + "' is " + i + " characters long")); // the consequence captures all the joined variables positionally
}
}
As you define rules using defineRules
method, you can execute the rules, of course, without having to define them using DRL.
public void helloWorld() {
HelloWorldUnit unit = new HelloWorldUnit();
unit.getStrings().add("Hello World");
RuleUnitInstance<HelloWorldUnit> unitInstance = RuleUnitProvider.get().createRuleUnitInstance(unit);
assertThat(unitInstance.fire()).isEqualTo(2);
assertThat(unit.getResults()).containsExactlyInAnyOrder("it worked!", "it also worked with HELLO WORLD");
unit.getResults().clear();
unit.getInts().add(11);
assertThat(unitInstance.fire()).isEqualTo(1);
assertThat(unit.getResults()).containsExactly("String 'Hello World' is 11 characters long");
unitInstance.close();
}
You can find various test cases under https://github.com/kiegroup/drools/tree/main/drools-ruleunits/drools-ruleunits-dsl
Using a KIE scanner to monitor and update KIE containers
The KIE scanner in Drools monitors your Maven repository for new SNAPSHOT
versions of your Drools project and then deploys the latest version of the project to a specified KIE container. You can use a KIE scanner in a development environment to maintain your Drools project deployments more efficiently as new versions become available.
For production environments, do not use a KIE scanner with |
-
The
kie-ci.jar
file is available on the class path of your Drools project.
-
In the relevant
.java
class in your project, register and start the KIE scanner as shown in the following example code:Registering and starting a KIE scanner for a KIE containerimport org.kie.api.KieServices; import org.kie.api.builder.ReleaseId; import org.kie.api.runtime.KieContainer; import org.kie.api.builder.KieScanner; ... KieServices kieServices = KieServices.Factory.get(); ReleaseId releaseId = kieServices .newReleaseId("com.sample", "my-app", "1.0-SNAPSHOT"); KieContainer kContainer = kieServices.newKieContainer(releaseId); KieScanner kScanner = kieServices.newKieScanner(kContainer); // Start KIE scanner for polling the Maven repository every 10 seconds (10000 ms) kScanner.start(10000L);
In this example, the KIE scanner is configured to run with a fixed time interval. The minimum KIE scanner polling interval is 1 millisecond (ms) and the maximum polling interval is the maximum value of the data type
long
. A polling interval of 0 or less results in ajava.lang.IllegalArgumentException: pollingInterval must be positive
error. You can also configure the KIE scanner to run on demand by invoking thescanNow()
method.The project group ID, artifact ID, and version (GAV) settings in the example are defined as
com.sample:my-app:1.0-SNAPSHOT
. The project version must contain the-SNAPSHOT
suffix to enable the KIE scanner to retrieve the latest build of the specified artifact version. If you change the snapshot project version number, such as increasing to1.0.1-SNAPSHOT
, then you must also update the version in the GAV definition in your KIE scanner configuration. The KIE scanner does not retrieve updates for projects with static versions, such ascom.sample:my-app:1.0
. -
In the
settings.xml
file of your Maven repository, set theupdatePolicy
configuration toalways
to enable the KIE scanner to function properly:<profile> <id>my-nexus-env</id> <repositories> <repository> <id>my-nexus</id> <name>My Nexus repository</name> <url>http://repository.example.org/nexus/content/groups/public/</url> <layout>default</layout> <releases> <enabled>true</enabled> <updatePolicy>always</updatePolicy> </releases> <snapshots> <enabled>true</enabled> <updatePolicy>always</updatePolicy> </snapshots> </repository> </repositories> </profile>
After the KIE scanner starts polling, if the KIE scanner detects an updated version of the
SNAPSHOT
project in the specified KIE container, the KIE scanner automatically downloads the new project version and triggers an incremental build of the new project. From that moment, all of the newKieBase
andKieSession
objects that were created from the KIE container use the new project version.
Security Manager
Starting with JDK17, the Java Platform has deprecated the Security Manager for removal; consequently, this Drools feature may become unsupported in the future. For more information: https://openjdk.org/jeps/411 |
The KIE engine is a platform for the modelling and execution of business behavior, using a multitude of declarative abstractions and metaphors, like rules, processes, decision tables and etc.
Many times, the authoring of these metaphors is done by third party groups, be it a different group inside the same company, a group from a partner company, or even anonymous third parties on the internet.
Rules and Processes are designed to execute arbitrary code in order to do their job, but in such cases it might be necessary to constrain what they can do.
For instance, it is unlikely a rule should be allowed to create a classloader (what could open the system to an attack) and certainly it should not be allowed to make a call to System.exit()
.
The Java Platform provides a very comprehensive and well defined security framework that allows users to define policies for what a system can do. The KIE platform leverages that framework and allow application developers to define a specific policy to be applied to any execution of user provided code, be it in rules, processes, work item handlers and etc.
How to define a KIE Policy
Rules and processes can run with very restrict permissions, but the engine itself needs to perform many complex operations in order to work. Examples are: it needs to create classloaders, read system properties, access the file system, etc.
Once a security manager is installed, though, it will apply restrictions to all the code executing in the JVM according to the defined policy. For that reason, KIE allows the user to define two different policy files: one for the engine itself and one for the assets deployed into and executed by the engine.
One easy way to setup the environment is to give the engine itself a very permissive policy, while providing a constrained policy for rules and processes.
Policy files follow the standard policy file syntax as described in the Java documentation. For more details, see:
A permissive policy file for the engine can look like the following:
grant {
permission java.security.AllPermission;
}
An example security policy for rules could be:
grant {
permission java.util.PropertyPermission "*", "read";
permission java.lang.RuntimePermission "accessDeclaredMembers";
}
Please note that depending on what the rules and processes are supposed to do, many more permissions might need to be granted, like accessing files in the filesystem, databases, etc.
In order to use these policy files, all that is necessary is to execute the application with these files as parameters to the JVM. Three parameters are required:
Parameter | Meaning |
---|---|
-Djava.security.manager |
Enables the security manager |
-Djava.security.policy=<jvm_policy_file> |
Defines the global policy file to be applied to the whole application, including the engine |
-Dkie.security.policy=<kie_policy_file> |
Defines the policy file to be applied to rules and processes |
For instance:
java -Djava.security.manager -Djava.security.policy=global.policy -Dkie.security.policy=rules.policy
foo.bar.MyApp
When executing the engine inside a container, use your container’s documentation to find out how to configure the Security Manager and how to define the global security policy.
Define the kie security policy as described above and set the |
Please note that unless a Security Manager is configured, the |
A Security Manager has a high performance impact in the JVM. Applications with strict performance requirements are strongly discouraged of using a Security Manager. An alternative is the use of other security procedures like the auditing of rules/processes before testing and deployment to prevent malicious code from being deployed to the environment. |